CameraInfo
CameraInfo
interface contains information of a camera.
Getting Available CameraInfo
s​
We can retrieve CameraInfo
instances of our device's available cameras by using getAvailableCameraInfos()
method. However, if we are limiting the available cameras with CameraXConfig
, then this method will only return the selected cameras.
import android.content.Context
import android.util.Log
import androidx.camera.lifecycle.ProcessCameraProvider
val provider = ProcessCameraProvider.getInstance(
context,
).get()
Log.i("TAG", provider.availableCameraInfos.toString())
Camera Zoom​
Getting Zoom State​
After retrieving CameraInfo
instance, we can get a LiveData
of the camera's zoom state. The LiveData will be updated whenever the zoom state has been changed. This includes whenever application uses setZoomRatio()
and setLinearZoom()
methods. The zoom state can also change anytime a camera starts up, for example when a UseCase
is bound to it.
import android.util.Log
import androidx.camera.core.CameraInfo
import androidx.camera.core.ZoomState
import androidx.lifecycle.LifecycleOwner
// val cameraInfo = ...
cameraInfo.zoomState.observe(lifecycleOwner) { zoomState: ZoomState ->
Log.i("zoomState", zoomState.toString())
}
The ZoomState
interface contains several information that could be useful for us:
Method | Description |
---|---|
getLinearZoom() | Returns the linearZoom which is in range [0..1]. LinearZoom 0 represents the minimum zoom while linearZoom 1.0 represents the maximum zoom. |
getZoomRatio() | Returns the zoom ratio. The value is 1.0 by default. |
getMinZoomRatio() | Returns the minimum zoom ratio. Typically 1.0, but can be less than 1.0 if the camera device supports zoom-out (only on android 11 or later). |
getMaxZoomRatio() | Returns the maximum zoom ratio. |
Camera Torch/Flashlight​
Checking Whether Camera Has Torch​
We can check whether a camera has a torch/flashlight unit by using hasFlashUnit()
method:
import androidx.camera.core.CameraInfo
// val cameraInfo = ...
val hasFlash: Boolean = cameraInfo.hasFlashUnit()
Getting Torch State​
We can also retrieve a LiveData
of the camera's torch state by using getTorchState()
method.
import android.util.Log
import androidx.camera.core.CameraInfo
import androidx.lifecycle.LifecycleOwner
// val cameraInfo = ...
cameraInfo.torchState.observe(lifecycleOwner) { torchState: Int ->
Log.i("torchState", torchState.toString())
}
There are only 2 possible torch states:
Torch state | Description |
---|---|
OFF | Torch is off. |
ON | Torch is on. |
Alternatively, CameraController
class also has the exact same method for this purpose, i.e. getTorchState()
.
Camera State​
Getting Camera State​
With CameraInfo
instance, we can retrieve a LiveData
of the camera's state by using getCameraState()
method:
import android.util.Log
import androidx.camera.core.CameraInfo
import androidx.camera.core.CameraState
import androidx.lifecycle.LifecycleOwner
// val cameraInfo = ...
cameraInfo.cameraState.observe(lifecycleOwner) { cameraState: CameraState ->
Log.i("cameraState", cameraState.toString())
}
Currently, these are the possible camera states:
Camera State | Description |
---|---|
CLOSED | Represents a state where the camera device is closed. The camera is initially in this state. Developers can rely on this state to be notified of when the camera device is actually closed, and then use this signal to free up camera resources, or start the camera device with another camera client. |
OPENING | Represents a state where the camera device is currently opening. Developers can rely on this state to be aware of when the camera is actively attempting to open the camera device, this allows them to communicate it to their users through the UI. |
OPEN | Represents a state where the camera device is open. Developers can rely on this state to be notified of when the camera device is actually ready for use, and can then set up camera dependent resources, especially if they're heavyweight. |
CLOSING | Represents a state where the camera device is currently closing. Developers can rely on this state to be aware of when the camera device is actually in the process of closing. this allows them to communicate it to their users through the UI. |
PENDING_OPEN | Represents a state where the camera is waiting for a signal to attempt to open the camera device. Developers may rely on this state to close any other open cameras in the app, or request their user close an open camera in another app. |
Here's a chart that illustrates the events that triggers the state changes:
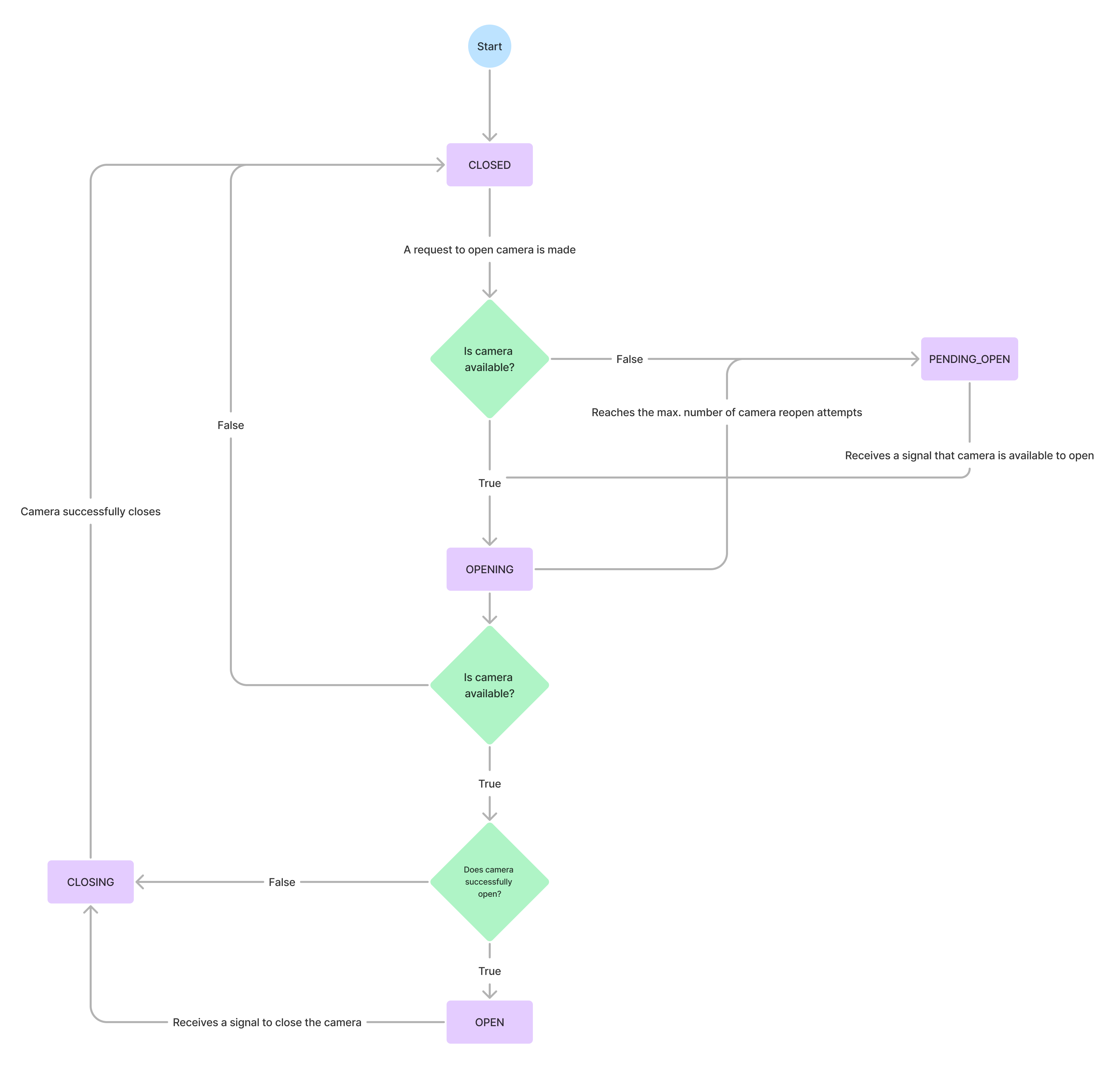