Logging
Log
class contains methods that developers can use for writing logs. You can view these logs, for example, in the Logcat.
Logging Properties​
Logging Verbosity​
Here are the 6 levels of verbosity in Android logging from highest to lowest:
Verbosity | Level |
---|---|
ASSERT | 7 (Highest) |
ERROR | 6 |
WARN | 5 |
INFO | 4 |
DEBUG | 3 |
VERBOSE | 2 (Lowest) |
Here's how each level will look like when you view them in Logcat:
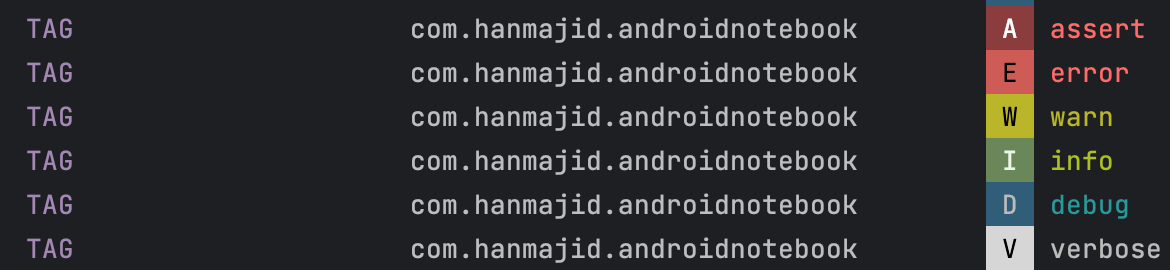
Logging Tag​
Tag is used to identify the source of the log message. It usually identifies the class or activity where the log occurs. You can also set this value to null
if you don't need it.
Logging Tag Best Practices​
A good convention for using logging tag is by declaring TAG
constant in your class:
class MyAwesomeClass {
companion object {
private const val TAG = "MyAwesomeClass"
}
}
And then using it when you're logging something:
import android.util.Log
class MyAwesomeClass {
fun myAwesomeMethod() {
Log.d(TAG, "Something is happening here")
}
}
It is also a good practice for the logging tag's length to not exceed 23 characters. There are instances of error that happened when developers used tag that is too long.
Logging Methods​
High-Level Method​
Log
class provides some high-level static methods that we can use for logging based on its level of verbosity:
Verbosity | Logging Method |
---|---|
ASSERT | - |
ERROR | Log.e() |
WARN | Log.w() |
INFO | Log.i() |
DEBUG | Log.d() |
VERBOSE | Log.v() |
Each of these methods have at least 1 other signature variation, where we can also specify an Exception
to log.
Here's an example of how to use these methods:
import android.util.Log
Log.e(TAG, "This is an error log")
Log.e(TAG, "This is an error log", Exception("This is my exception"))
println
​
Log
class also provides low-level logging method called println()
where we can specify directly the level of verbosity as an argument:
import android.util.Log
Log.println(Log.VERBOSE, TAG, "Something is happening here")
WTF (What a Terrible Failure)​
There are also some special logging methods that we can use called Log.wtf()
. This method should be used to log a condition that should not happen. The error will always be logged at level ASSERT
(or ERROR
based on my experience) with the call stack. Depending on the system configuration, a report may be added to the DropboxManager
and/or the process may be terminated immediately with an error dialog.
import android.util.Log
Log.wtf(TAG, "This should not happen!")
Getting Stack Trace String​
Log
class also has a useful method for retrieving a loggable stack trace from a Throwable/Exception. To do this, simply use getStackTraceString()
method like this:
import android.util.Log
val myStackTrace = Log.getStackTraceString(Exception("This is my exception"))
Checking Whether Tag is Loggable​
Log
class also provides isLoggable()
method that we can use to check whether a log with the specified tag is loggable at the specified level.
By default, all tag will have level INFO
. This means that any level above and including INFO
will be logged:
import android.util.Log
Log.v(TAG, "verbose: " + Log.isLoggable(TAG, Log.VERBOSE).toString()) // false
Log.d(TAG, "debug: " + Log.isLoggable(TAG, Log.DEBUG).toString()) // false
Log.i(TAG, "info: " + Log.isLoggable(TAG, Log.INFO).toString()) // true
Log.w(TAG, "warn: " + Log.isLoggable(TAG, Log.WARN).toString()) // true
Log.e(TAG, "error: " + Log.isLoggable(TAG, Log.ERROR).toString()) // true
Log.wtf(TAG, "assert: " + Log.isLoggable(TAG, Log.ASSERT).toString()) // true
Although, as we can see above, isLoggable()
method returns false for Log.VERBOSE
and Log.DEBUG
, we can still use Log.v()
and Log.d()
methods unless we explicitly set the tag loggable level ourselves.
Setting Tag Loggable Level​
We can explicitly set a tag's loggable level by using adb:
# Set "MyAwesomeClass" tag loggable level to VERBOSE.
adb shell setprop log.tag.MyAwesomeClass VERBOSE
We can set our tag's loggable level to either VERBOSE
, DEBUG
, INFO
, WARN
, ERROR
, or ASSERT
.
We can also get current tag's loggable level and reset it by using these commands:
# Get current "MyAwesomeClass" tag loggable level.
adb shell getprop log.tag.MyAwesomeClass
# Reset "MyAwesomeClass" tag loggable level.
adb shell setprop log.tag.MyAwesomeClass \"\"