Live Wallpaper Basic Operations
Live wallpaper is interactive and animated Android home screen wallpaper/background introduced in Android 2.1.
As a developer, there are some basic operations that we can do regarding live wallpapers:
1. Launching Live Wallpaper Chooser Activity​
With ACTION_LIVE_WALLPAPER_CHOOSER
, we can launch an Activity to select live wallpapers that are available in our device as active wallpaper.
import android.app.WallpaperManager
import android.content.Context
import android.content.Intent
import androidx.core.content.ContextCompat.startActivity
// Launch live wallpaper chooser Activity
startActivity(context, Intent(WallpaperManager.ACTION_LIVE_WALLPAPER_CHOOSER), null)
Here's what happen when we launch the Activity:
2. Listing Available Live Wallpapers​
We can get the list of all live wallpapers that are available in a device by querying WallpaperService
.
First, we need to declare query intent filter with the action "android.service.wallpaper.WallpaperService"
inside AndroidManifest.xml
:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<application>
</application>
<queries>
<intent>
<action android:name="android.service.wallpaper.WallpaperService" />
</intent>
</queries>
</manifest>
Then we need to call queryIntentServices()
method with WallpaperService.SERVICE_INTERFACE
intent:
import android.app.WallpaperInfo
import android.content.Context
import android.content.Intent
import android.content.pm.PackageManager
import android.service.wallpaper.WallpaperService
// Get available live wallpapers
val wallpapers: List<WallpaperInfo> = context.packageManager.queryIntentServices(
Intent(WallpaperService.SERVICE_INTERFACE),
PackageManager.GET_META_DATA,
).map {
WallpaperInfo(context, it)
}
Finally, we can use the properties available in WallpaperInfo
class to get the relevant live wallpaper information. For example, the Activity below displays the thumbnail, label, and description of the live wallpapers.
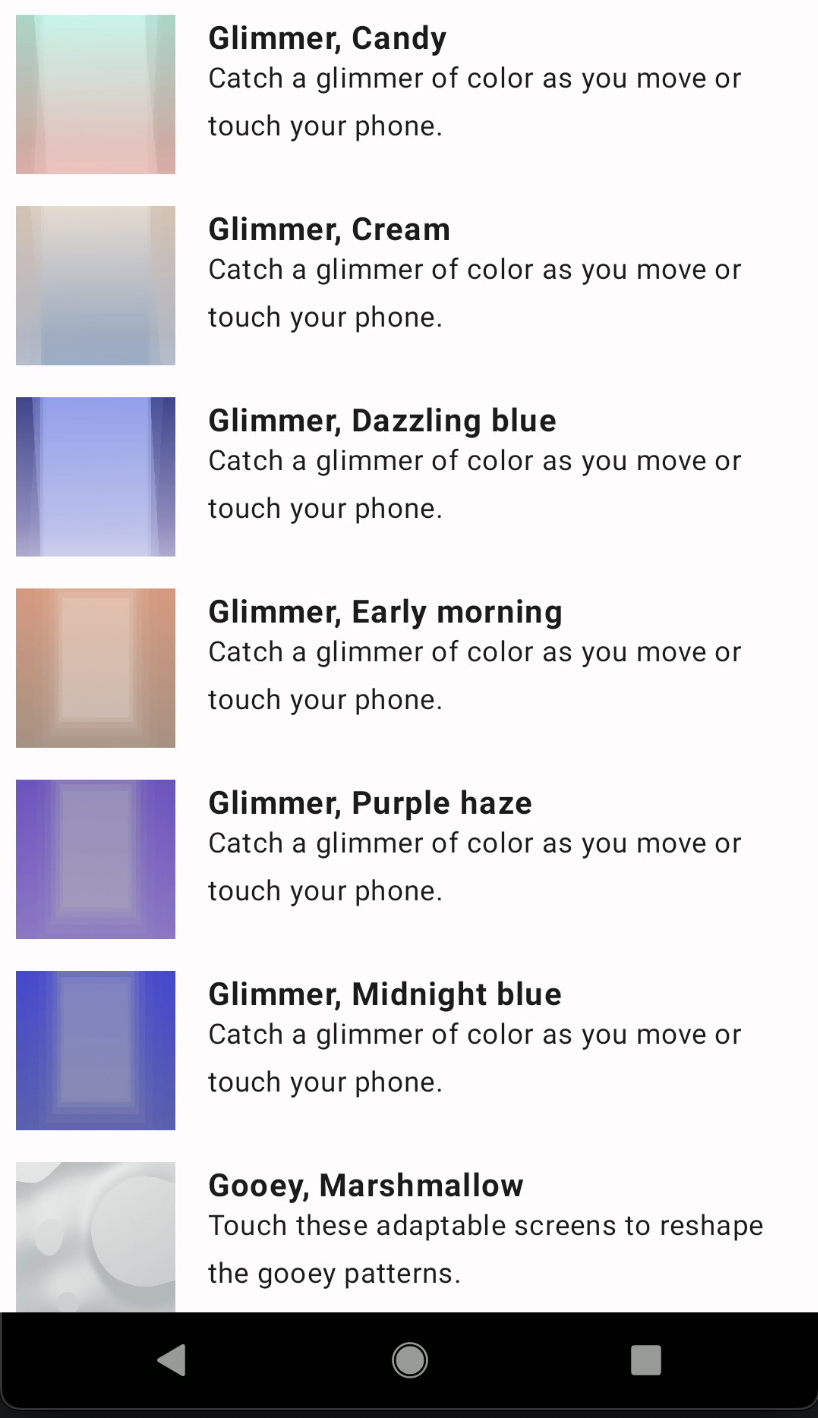
3. Changing Live Wallpaper​
With ACTION_CHANGE_LIVE_WALLPAPER
, we can change the current live wallpaper with the one we specified.
import android.app.WallpaperInfo
import android.app.WallpaperManager
import android.content.Context
import android.content.Intent
import androidx.core.content.ContextCompat.startActivity
// Change live wallpaper
// val liveWallpaper: WallpaperInfo = ...
startActivity(
context,
Intent(WallpaperManager.ACTION_CHANGE_LIVE_WALLPAPER).apply {
putExtra(
WallpaperManager.EXTRA_LIVE_WALLPAPER_COMPONENT,
liveWallpaper.component
)
},
null,
)
Here's what happen when we launch the Activity: