Notification Channel Groups
Notification channel (or notification category) is introduced in Android 8.0 (API level 26). You can group these notification channels together in a collection called notification channel group.
Here's an example of a notification channel group in Google application:
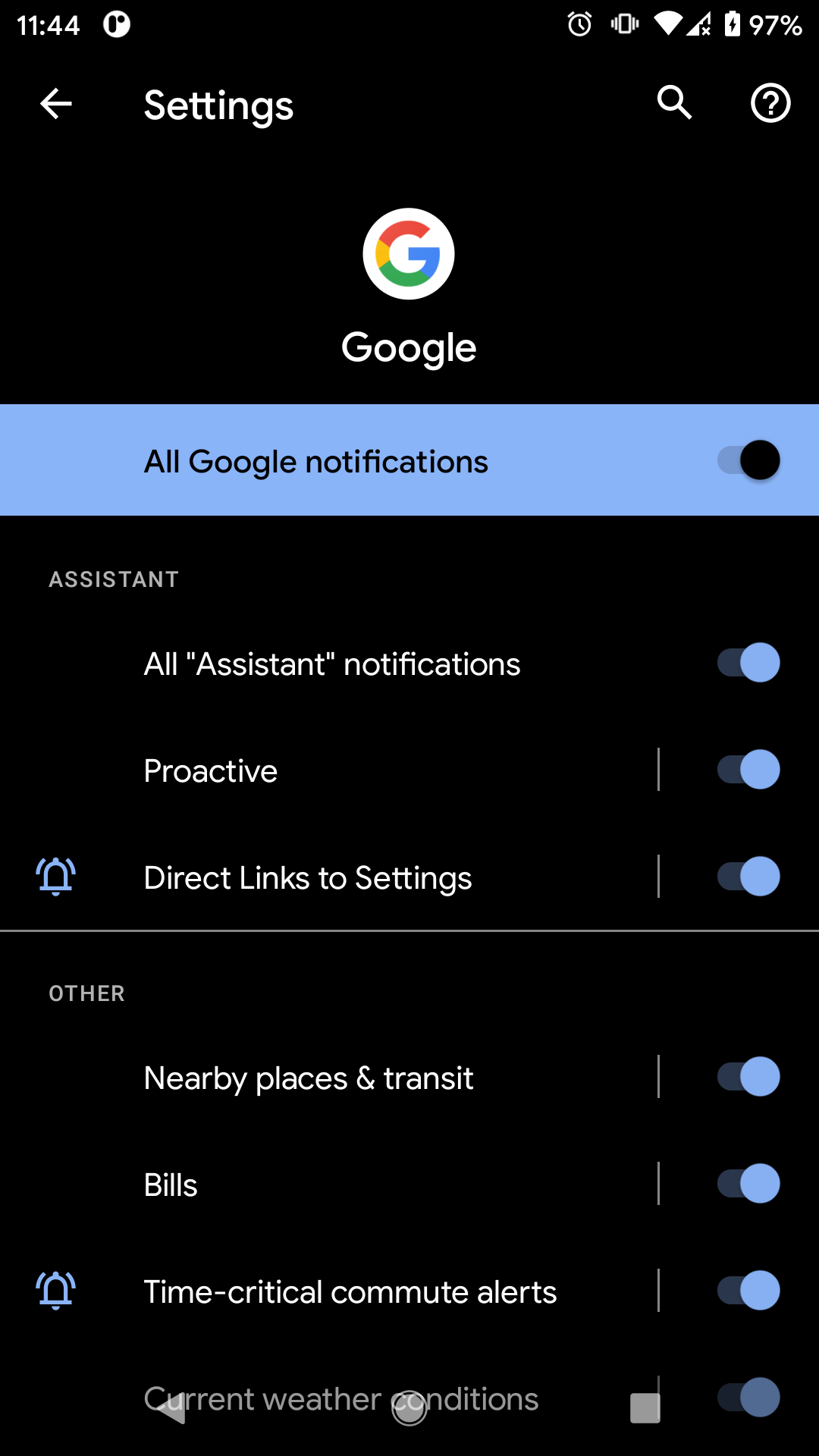
The notification channel is "Assistant". Notification channels that are not assigned to a group will be located in the "Other" default group.
By using notification channel groups, user can block/unblock a group directly. They don't have to block/unblock notification channels individually.
Basic Usages​
There are a few things you can do related to notification channel groups as developers.
Creating Notification Channel Group​
You can create a notification channel group for your application programmatically by using createNotificationChannelGroup()
method. Or alternatively, if you want to create multiple notification channel groups simultaneously, you can use createNotificationChannelGroups()
method instead.
import android.app.NotificationChannelGroup
import android.app.NotificationManager
import android.content.Context
import android.os.Build
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
val notificationManager = context.getSystemService(NotificationManager::class.java)
val group1 = NotificationChannelGroup(
"my-channel-group",
"My Channel Group"
)
notificationManager.createNotificationChannelGroup(group1)
}
Assigning Notification Channel to a Group​
After creating a notification channel group, you can assign a notification channel to it by using the setGroup()
method:
import android.app.NotificationChannel
import android.app.NotificationManager
import android.content.Context
import android.os.Build
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
val channelId = "channel-latest-news"
val name = "Latest News & Info"
val channel = NotificationChannel(
channelId,
name,
NotificationManager.IMPORTANCE_DEFAULT,
).apply {
description = "Notify user about the latest news & information about Android Notebook."
group = group1.id
}
val notificationManager = context.getSystemService(NotificationManager::class.java)
notificationManager.createNotificationChannel(channel)
}
Getting All Application Notification Channel Groups​
You can retrieve all of your application's notification channel groups by using getNotificationChannelGroups()
method:
import android.app.NotificationManager
import android.content.Context
import android.os.Build
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
val notificationManager = context.getSystemService(NotificationManager::class.java)
val groups = notificationManager.notificationChannelGroups
}
Getting Notification Channel Group by ID​
Starting in Android 9 (API level 28), you can retrieve a notification channel group by its group id by using getNotificationChannelGroup()
method:
import android.app.NotificationManager
import android.content.Context
import android.os.Build
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.P) {
val groupId = "my-channel-group"
val notificationManager = context.getSystemService(NotificationManager::class.java)
val group = notificationManager.getNotificationChannelGroup(groupId)
}
If the notification channel group doesn't exist or has been deleted, this method will return null
.
Deleting Notification Channel Group​
You can delete your application's notification channel group by using deleteNotificationChannelGroup()
method:
import android.app.NotificationManager
import android.content.Context
import android.os.Build
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
val groupId = "my-channel-group"
val notificationManager = context.getSystemService(NotificationManager::class.java)
notificationManager.deleteNotificationChannelGroup(groupId)
}
Listening to Notification Channel Group Block State​
You can also listen to a broadcast intent whenever your application's notification channel group is blocked/unblocked by the user. To do this, you can create a broadcast receiver and listen to ACTION_NOTIFICATION_CHANNEL_GROUP_BLOCK_STATE_CHANGED
intent action:
package com.hanmajid.androidnotebook
import android.app.NotificationManager
import android.content.BroadcastReceiver
import android.content.Context
import android.content.Intent
import android.os.Build
import android.util.Log
/**
* Simple broadcast receiver that listens to
* [NotificationManager.ACTION_NOTIFICATION_CHANNEL_GROUP_BLOCK_STATE_CHANGED] intent action.
*/
class NotificationChannelGroupBlockStateBroadcastReceiver : BroadcastReceiver() {
override fun onReceive(context: Context, intent: Intent) {
val groupId = if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.P) {
intent.getStringExtra(NotificationManager.EXTRA_NOTIFICATION_CHANNEL_GROUP_ID)
} else {
null
}
val isBlocked = if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.P) {
intent.getBooleanExtra(NotificationManager.EXTRA_BLOCKED_STATE, false)
} else {
null
}
Log.i("TAG", "groupId: $groupId")
Log.i("TAG", "isBlocked: $isBlocked")
}
}
Then you can register the broadcast receiver within your context:
import android.app.NotificationManager
import android.content.Context
import android.content.IntentFilter
import android.os.Build
import androidx.core.content.ContextCompat
// Initialize broadcast receiver within your context.
val broadcastReceiver = NotificationChannelGroupBlockStateBroadcastReceiver()
// Register broadcast receiver to [context].
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.P) {
ContextCompat.registerReceiver(
context,
broadcastReceiver,
IntentFilter(NotificationManager.ACTION_NOTIFICATION_CHANNEL_GROUP_BLOCK_STATE_CHANGED),
ContextCompat.RECEIVER_NOT_EXPORTED,
)
}
References​
- Create a notification channel group | Android Developers
ACTION_NOTIFICATION_CHANNEL_GROUP_BLOCK_STATE_CHANGED
| Android DeveloperscreateNotificationChannelGroup()
| Android DeveloperscreateNotificationChannelGroups()
| Android DevelopersdeleteNotificationChannelGroup()
| Android DevelopersgetNotificationChannelGroup()
| Android DevelopersgetNotificationChannelGroups()
| Android DeveloperssetGroup()
| Android Developers