Notification Full Screen Intents
Instead of posting notification, it is possible to launch an intent instead by using setFullScreenIntent()
method.
Basic Usage​
There are several things we can do related to notification full screen intents.
1. Opening Application Full Screen Intent Setting Page​
Starting in Android 14, to open a page to manage our application's full screen intent's settings, we can use ACTION_MANAGE_APP_USE_FULL_SCREEN_INTENT
:
import android.content.Context
import android.content.Intent
import android.net.Uri
import android.os.Build
import android.provider.Settings
import androidx.core.content.ContextCompat.startActivity
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.UPSIDE_DOWN_CAKE) {
startActivity(
context,
Intent(Settings.ACTION_MANAGE_APP_USE_FULL_SCREEN_INTENT).apply {
data = Uri.parse("package:${context.packageName}")
},
null,
)
}
Running this code will open setting page similar to this one:
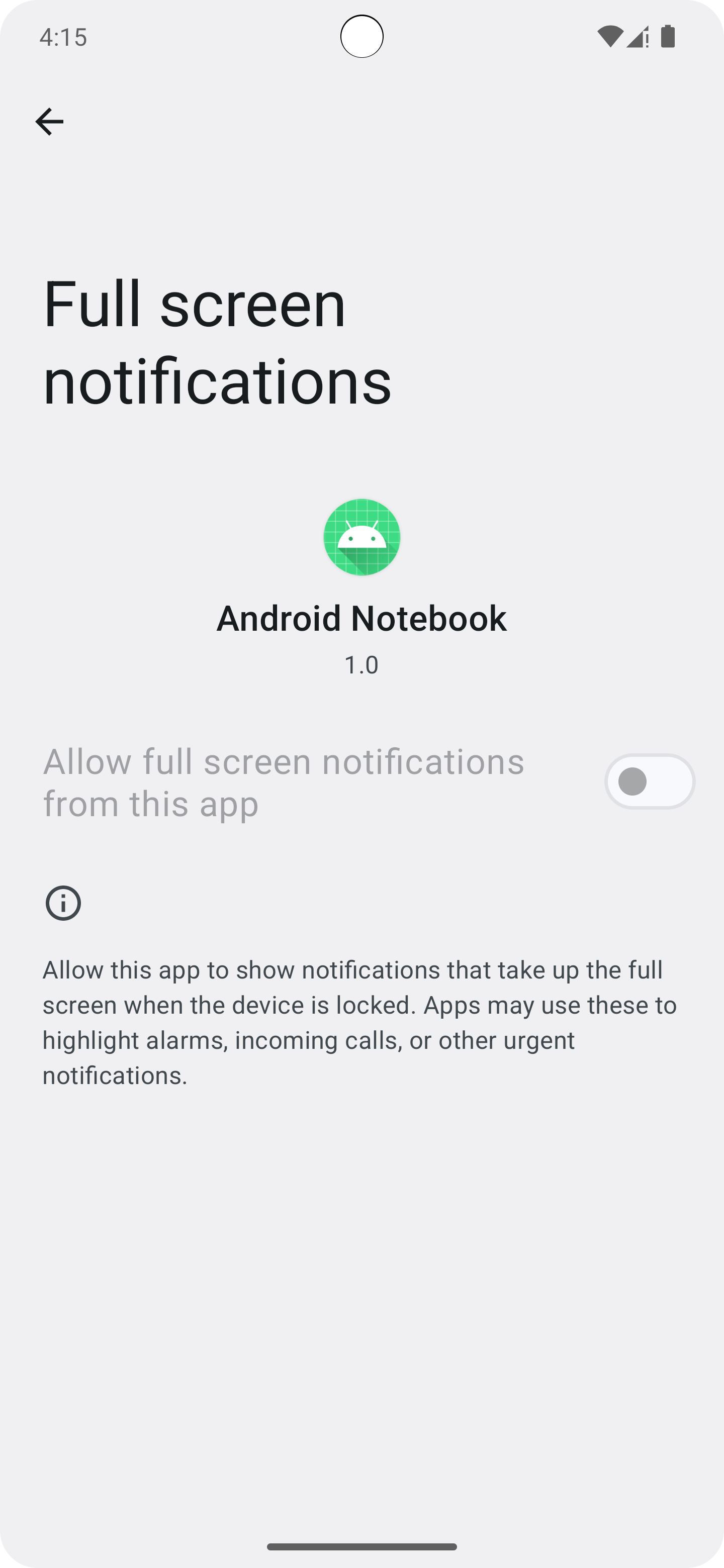
By default, the toggle is disabled. If you want to enable the toggle, you need to add USE_FULL_SCREEN_INTENT
permission to AndroidManifest.xml
file:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<!-- Add this permission: -->
<uses-permission android:name="android.permission.USE_FULL_SCREEN_INTENT" />
<application>
</application>
</manifest>
If the permission is added, the toggle will be enabled (allowed by default):
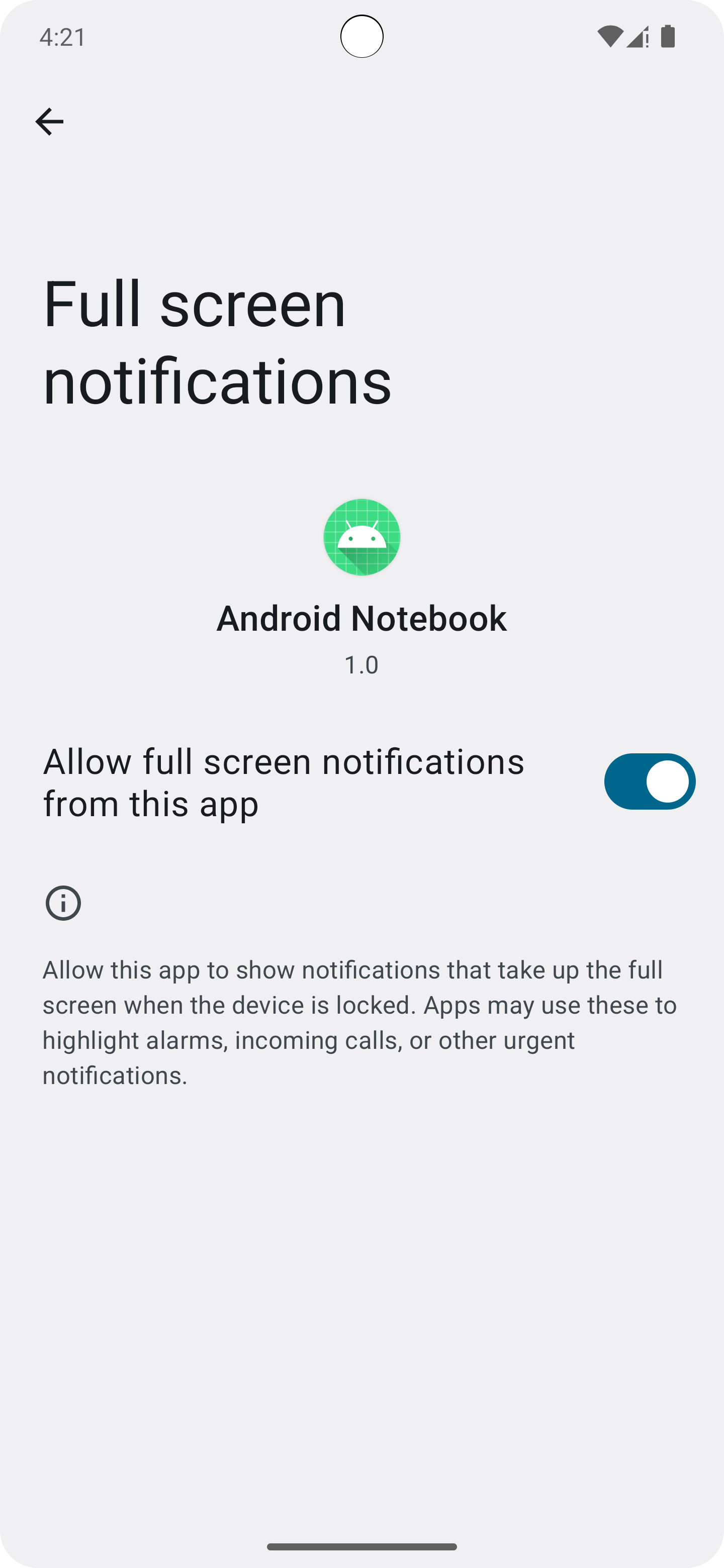
2. Checking Whether App Can Use Full Screen Intent​
Starting from Android 14, we can also check whether our app is allowed to use notification full screen intent by using canUseFullScreenIntent()
method:
import android.app.NotificationManager
import android.content.Context
import android.os.Build
import android.util.Log
val notificationManager = context.getSystemService(NotificationManager::class.java)
val canUseFullScreenIntent = if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.UPSIDE_DOWN_CAKE) {
notificationManager.canUseFullScreenIntent()
} else {
null
}
Log.i("TAG", "App can use full screen intent: $canUseFullScreenIntent")